The Graph: Fixing Web3 data querying
This time we will take a closer look at The Graph which essentially became part of the standard stack for developing dapps in the last year. Let's first see how we would do things the traditional way...
Without The Graph...
So let's go with a simple example for illustration purposes. We all like games, so imagine a simple game with users placing bets:
1pragma solidity 0.7.1;23contract Game {4 uint256 totalGamesPlayerWon = 0;5 uint256 totalGamesPlayerLost = 0;6 event BetPlaced(address player, uint256 value, bool hasWon);78 function placeBet() external payable {9 bool hasWon = evaluateBetForPlayer(msg.sender);1011 if (hasWon) {12 (bool success, ) = msg.sender.call{ value: msg.value * 2 }('');13 require(success, "Transfer failed");14 totalGamesPlayerWon++;15 } else {16 totalGamesPlayerLost++;17 }1819 emit BetPlaced(msg.sender, msg.value, hasWon);20 }21}Εμφάνιση όλωνΑντιγραφή
Now let's say in our dapp, we want to display total bets, the total games lost/won and also update it whenever someone plays again. The approach would be:
- Fetch
totalGamesPlayerWon
. - Fetch
totalGamesPlayerLost
. - Subscribe to
BetPlaced
events.
We can listen to the event in Web3(opens in a new tab) as shown on the right, but it requires handling quite a few cases.
1GameContract.events.BetPlaced({2 fromBlock: 03}, function(error, event) { console.log(event); })4.on('data', function(event) {5 // event fired6})7.on('changed', function(event) {8 // event was removed again9})10.on('error', function(error, receipt) {11 // tx rejected12});Εμφάνιση όλωνΑντιγραφή
Now this is still somewhat fine for our simple example. But let's say we want to now display the amounts of bets lost/won only for the current player. Well we're out of luck, you better deploy a new contract that stores those values and fetch them. And now imagine a much more complicated smart contract and dapp, things can get messy quickly.
You can see how this is not optimal:
- Doesn't work for already deployed contracts.
- Extra gas costs for storing those values.
- Requires another call to fetch the data for an Ethereum node.
Now let's look at a better solution.
Let me introduce you to GraphQL
First let's talk about GraphQL, originally designed and implemented by Facebook. You might be familiar with the traditional REST API model. Now imagine instead you could write a query for exactly the data that you wanted:
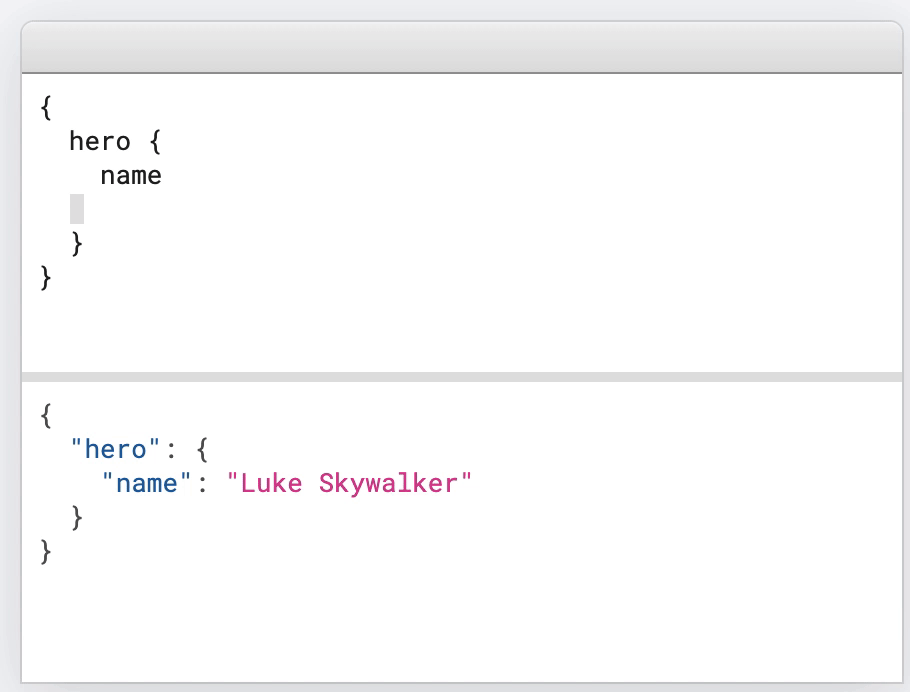
The two images pretty much capture the essence of GraphQL. With the query on the right we can define exactly what data we want, so there we get everything in one request and nothing more than exactly what we need. A GraphQL server handles the fetching of all data required, so it is incredibly easy for the frontend consumer side to use. This is a nice explanation(opens in a new tab) of how exactly the server handles a query if you're interested.
Now with that knowledge, let's finally jump into blockchain space and The Graph.
What is The Graph?
A blockchain is a decentralized database, but in contrast to what's usually the case, we don't have a query language for this database. Solutions for retrieving data are painful or completely impossible. The Graph is a decentralized protocol for indexing and querying blockchain data. And you might have guessed it, it's using GraphQL as query language.
Examples are always the best to understand something, so let's use The Graph for our GameContract example.
How to create a Subgraph
The definition for how to index data is called subgraph. It requires three components:
- Manifest (
subgraph.yaml
) - Schema (
schema.graphql
) - Mapping (
mapping.ts
)
Manifest (subgraph.yaml
)
The manifest is our configuration file and defines:
- which smart contracts to index (address, network, ABI...)
- which events to listen to
- other things to listen to like function calls or blocks
- the mapping functions being called (see
mapping.ts
below)
You can define multiple contracts and handlers here. A typical setup would have a subgraph folder inside the Truffle/Hardhat project with its own repository. Then you can easily reference the ABI.
For convenience reasons you also might want to use a template tool like mustache. Then you create a subgraph.template.yaml
and insert the addresses based on the latest deployments. For a more advanced example setup, see for example the Aave subgraph repo(opens in a new tab).
And the full documentation can be seen here(opens in a new tab).
1specVersion: 0.0.12description: Placing Bets on Ethereum3repository: - GitHub link -4schema:5 file: ./schema.graphql6dataSources:7 - kind: ethereum/contract8 name: GameContract9 network: mainnet10 source:11 address: '0x2E6454...cf77eC'12 abi: GameContract13 startBlock: 617524414 mapping:15 kind: ethereum/events16 apiVersion: 0.0.117 language: wasm/assemblyscript18 entities:19 - GameContract20 abis:21 - name: GameContract22 file: ../build/contracts/GameContract.json23 eventHandlers:24 - event: PlacedBet(address,uint256,bool)25 handler: handleNewBet26 file: ./src/mapping.tsΕμφάνιση όλων
Schema (schema.graphql
)
The schema is the GraphQL data definition. It will allow you to define which entities exist and their types. Supported types from The Graph are
- Bytes
- ID
- String
- Boolean
- Int
- BigInt
- BigDecimal
You can also use entities as type to define relationships. In our example we define a 1-to-many relationship from player to bets. The ! means the value can't be empty. The full documentation can be seen here(opens in a new tab).
1type Bet @entity {2 id: ID!3 player: Player!4 playerHasWon: Boolean!5 time: Int!6}78type Player @entity {9 id: ID!10 totalPlayedCount: Int11 hasWonCount: Int12 hasLostCount: Int13 bets: [Bet]!14}Εμφάνιση όλων
Mapping (mapping.ts
)
The mapping file in The Graph defines our functions that transform incoming events into entities. It is written in AssemblyScript, a subset of Typescript. This means it can be compiled into WASM (WebAssembly) for more efficient and portable execution of the mapping.
You will need to define each function named in the subgraph.yaml
file, so in our case we need only one: handleNewBet
. We first try to load the Player entity from the sender address as id. If it doesn't exist, we create a new entity and fill it with starting values.
Then we create a new Bet entity. The id for this will be event.transaction.hash.toHex() + "-" + event.logIndex.toString()
ensuring always a unique value. Using only the hash isn't enough as someone might be calling the placeBet function several times in one transaction via a smart contract.
Lastly we can update the Player entity with all the data. Arrays cannot be pushed to directly, but need to be updated as shown here. We use the id to reference the bet. And .save()
is required at the end to store an entity.
The full documentation can be seen here: https://thegraph.com/docs/en/developing/creating-a-subgraph/#writing-mappings(opens in a new tab). You can also add logging output to the mapping file, see here(opens in a new tab).
1import { Bet, Player } from "../generated/schema"2import { PlacedBet } from "../generated/GameContract/GameContract"34export function handleNewBet(event: PlacedBet): void {5 let player = Player.load(event.transaction.from.toHex())67 if (player == null) {8 // create if doesn't exist yet9 player = new Player(event.transaction.from.toHex())10 player.bets = new Array<string>(0)11 player.totalPlayedCount = 012 player.hasWonCount = 013 player.hasLostCount = 014 }1516 let bet = new Bet(17 event.transaction.hash.toHex() + "-" + event.logIndex.toString()18 )19 bet.player = player.id20 bet.playerHasWon = event.params.hasWon21 bet.time = event.block.timestamp22 bet.save()2324 player.totalPlayedCount++25 if (event.params.hasWon) {26 player.hasWonCount++27 } else {28 player.hasLostCount++29 }3031 // update array like this32 let bets = player.bets33 bets.push(bet.id)34 player.bets = bets3536 player.save()37}Εμφάνιση όλων
Using it in the Frontend
Using something like Apollo Boost, you can easily integrate The Graph in your React dapp (or Apollo-Vue). Especially when using React hooks and Apollo, fetching data is as simple as writing a single GraphQl query in your component. A typical setup might look like this:
1// See all subgraphs: https://thegraph.com/explorer/2const client = new ApolloClient({3 uri: "{{ subgraphUrl }}",4})56ReactDOM.render(7 <ApolloProvider client={client}>8 <App />9 </ApolloProvider>,10 document.getElementById("root")11)Εμφάνιση όλων
And now we can write for example a query like this. This will fetch us
- how many times current user has won
- how many times current user has lost
- a list of timestamps with all his previous bets
All in one single request to the GraphQL server.
1const myGraphQlQuery = gql`2 players(where: { id: $currentUser }) {3 totalPlayedCount4 hasWonCount5 hasLostCount6 bets {7 time8 }9 }10`1112const { loading, error, data } = useQuery(myGraphQlQuery)1314React.useEffect(() => {15 if (!loading && !error && data) {16 console.log({ data })17 }18}, [loading, error, data])Εμφάνιση όλων
But we're missing one last piece of the puzzle and that's the server. You can either run it yourself or use the hosted service.
The Graph server
Graph Explorer: The hosted service
The easiest way is to use the hosted service. Follow the instructions here(opens in a new tab) to deploy a subgraph. For many projects you can actually find existing subgraphs in the explorer(opens in a new tab).
Running your own node
Alternatively you can run your own node. Docs here(opens in a new tab). One reason to do this might be using a network that's not supported by the hosted service. The currently supported networks can be found here(opens in a new tab).
The decentralized future
GraphQL supports streams as well for newly incoming events. These are supported on the graph through Substreams(opens in a new tab) which are currently in open beta.
In 2021(opens in a new tab) The Graph began its transition to a decentralized indexing network. You can read more about the architecture of this decentralized indexing network here(opens in a new tab).
Two key aspects are:
- Users pay the indexers for queries.
- Indexers stake Graph Tokens (GRT).
Τελευταία επεξεργασία: , 11 Απριλίου 2024